Building a Multiplayer Backend: Accounts
Here at Wolfjaw Studios, we build game backends for a living. Over the last 5 years we have built partnerships with amazing publishers and studios. We have had the opportunity to work on titles like NBA2K, Destiny 2, League of Legends, Among Us, Apex Legends, and several unannounced titles. For more information on who we are, you can read The Story of Wolfjaw Studios and Raising the Bar with Catena Tools.
This article is the second in a series where we will discuss some of the basics of building out a multiplayer backend. Part 1: Authentication can be found here.
We’ll pick up where we left off in our last post and paint a better picture of what comes after authentication; accounts.
The Difference Between Authentication and Accounts
In part one of this series, we discussed authentication and authorization. The next piece of the puzzle in player management is accounts. While closely related, accounts differ significantly from authentication and authorization. First, let's briefly revisit the key differences between these concepts.
Authentication is used to verify an entity’s identity. In our case, this is a player.
Authorization is used to determine what that player is and is not allowed to do. For example, we may not allow a player to interact with certain game systems until they reach a certain level. Until that point, they are not authorized to hit certain endpoints.
Accounts are used to store various pieces of metadata about a player long-term.
Why Getting Accounts Right On Day One Is Important
If there is one thing you want to get right early, it’s your account system. Relatively speaking, migrating other systems (inventory, matchmaking, social features, leaderboards) to alternative implementations post-launch is a lot easier than migrating an account system. This is because the account system is the building block on which the rest of your backend is built.
If you launch your game, players begin to sign up and associate metadata with their account, and then you decide that the direction you chose is not meeting your needs, you now are faced with the tedious task of swapping a plane’s engine mid-flight. Because of this, it is strongly recommended that you take the following points into consideration when choosing how to build out your account system:
Types of Account Systems
There are various types of account systems you can choose for your game. The one you end up with will largely depend on a variety of factors. The first question to ask yourself is whether you’d like to lean on first party providers directly, to build something entirely from scratch, or to utilize a third party account solution.
First Party
Leaning on first party providers for your account system is the best option if you only plan to ship your game in one place. First party providers are where your game is shipped and/or played. These can include, but are not limited to:
- Apple, for iOS games
- Google, for Android games
- Steam, for PC games distributed through Steam
- Epic Game Store, for PC games distributed through the Epic Game Store
- Sony, for Playstation games
- Microsoft, for Xbox or PC Game Pass games
- Nintendo, for games shipped on Nintendo platforms
- Meta, for VR games
A good use case for when you’d want to utilize a first party provider for your account system is when you’re planning on only shipping your game on Steam. In this scenario, you can utilize Steamworks which provides methods for identifying a user. Down the road, when you want to build additional features into your game, you can continue to lean on Steam’s offering which includes features such as Matchmaking, Achievements, and User-Generated Content. Alternatively, if you don’t want to use Steamworks for anything other than player accounts, you can use its account system in isolation and write your other backend components custom by leaning on Steam’s session tickets functionality to verify a player’s identity without being the authority over their account. This use case is not unique to Steam, and may be what’s best for your game if you’re only shipping on one of the above listed platforms.
On the other hand, if you are planning on shipping your game through multiple providers, solely relying on their account system offerings is not sufficient without introducing some type of centralized account system. More on that in a bit.
Verdict: Only use a first party provider’s account system if you’re only planning on shipping your game with one provider.
A Quick Detour: How Centralized Account Systems Work At a High Level
If you’re planning on shipping your game across multiple first party providers and are planning on implementing cross-play or cross-progression features (now or at any point in the future), you are better off going with a third party accounts solution or building something custom. First, let’s define what we mean when we say cross-play and cross-progression.
Cross-play, sometimes referred to as cross-platform, allows players on different platforms to play together, including in multiplayer scenarios where they can interact across platform boundaries.
Cross-progression, sometimes referred to as cross-save, is when a single player can play a game on multiple platforms they own, logging in to the same central account on each platform and having their player state persist.
If you are to lean on first party providers alone, it is incredibly difficult to implement cross-play or cross progression features, as you will be juggling multiple account identifiers that all follow different formats. Let’s take matchmaking as an example:
While it’s possible to manage this, it becomes pretty difficult to do so as your backend feature set grows. This is where a centralized account system can be incredibly helpful, acting as a single source of truth for who a player is. With these systems, players still authenticate with first party providers, but you can think of their central account as a “keyring” with the various identity providers being “keys” that grant access.
Let’s assume we’re creating tables in a relational database to structure this.
CREATE TABLE accounts (
account_id VARCHAR NOT NULL PRIMARY KEY,
display_name VARCHAR NOT NULL
);
CREATE TABLE identity_providers (
accounts_account_id VARCHAR NOT NULL,
provider_account_id VARCHAR NOT NULL,
provider_type VARCHAR NOT NULL,
PRIMARY KEY (provider_account_id, provider_type),
CONSTRAINT account_identity_providers FOREIGN KEY (accounts_account_id) REFERENCES accounts (account_id)
);
Here, we create our own notion of an account. We keep track of every identity provider a player authenticates with along with some useful metadata about their account on that provider, such as the identity_providers.provider_account_id
to store the native account ID. We’ll rarely use this, and instead will use the accounts.account_id
as our source of truth across our backend. We end up with a schema that looks like this:
Here, our accounts
table is our “keyring.” We will use a player’s accounts.account_id
to reference that player in every subsequent backend feature we build. Our identity_providers
table can hold various “keys” on that keyring and contains some metadata about the identity provider’s notion of that player. We will talk more about how to manage keys on the keyring in just a moment, but for now you get a rough idea of how we are storing this information.
Now, if we revisit our matchmaking example, we can now utilize this single source of truth without needing to juggle multiple ID types to accomplish what you need.
Account Linking vs. Account Merging
In order to link multiple identities to a central account, an account linking or an account merging system needs to exist. First, let’s define each option.
Account Linking is the process of linking identity providers to a central account, or keyring. It is significantly less complex than account merging.
Account Merging is the process of merging two or more central accounts that have state associated with them. It is significantly more complex than account linking as there are many edge cases to consider.
Our recommended approach to account linking is to prompt a player when they first boot your game to determine whether or not they’ve created an account elsewhere before. If they have, you can kick off an account linking flow that will bring them through the process of attaching the new identity provider they’re using today with the central account they made previously. This console-friendly approach to account linking ensures a consistent experience for players looking to link their accounts no matter which platform they are on.
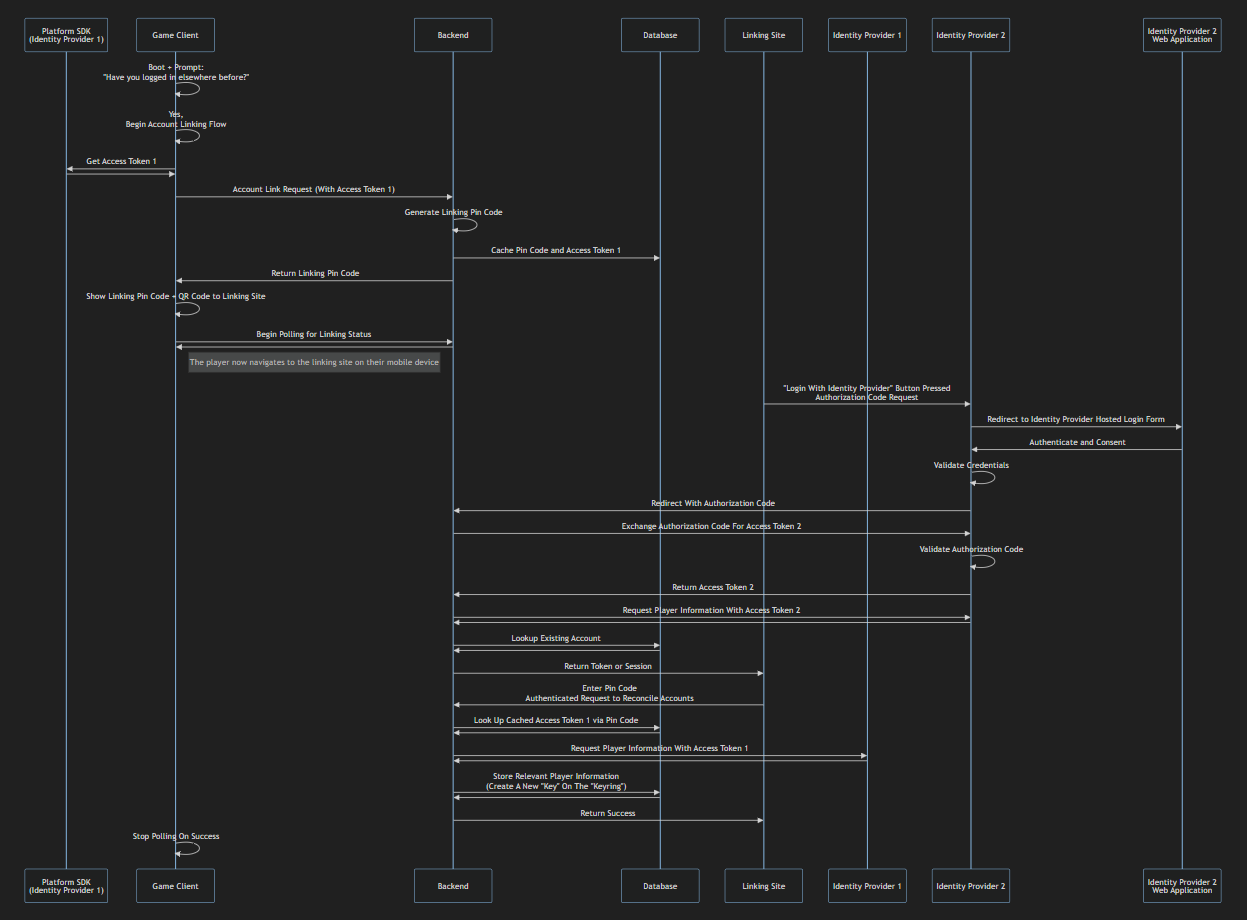
Once you have your base linking flow configured, it is also recommended to flesh out the “Linking Site” component shown above. It should also allow for players to manage the keys on their keyring outside of the main linking flow, giving them the opportunity to link/unlink identity providers from their central account as needed.
Sometimes, account linking itself will not be enough and you may need to merge two central accounts that each have a distinct state associated with them. This can happen if your account linking system is not easy to use and players make the mistake of creating multiple central accounts. Depending on the additional backend features you have in place, this process can be fraught with edge cases.
- What if each keyring has one key type (i.e. Steam) but they do not represent the same identity (different Steam accounts)? Which one do you keep?
- If a player has made progress on both accounts, how do you merge each inventory?
- If one account is a child account and the other is an adult account, how do you reconcile the two?
There is no one-size-fits-all approach to handling account merging. We typically recommend against implementing it if you can, but sometimes it’s a necessity.
Now that you have a better understanding of centralized account systems, let’s return to evaluating which type of account system is best for you.
Custom Centralized Accounts
The driving factor in choosing to develop a custom solution is how much experience your team has with these types of systems. If you have a lot of experience, and you have the capacity to develop, deploy, and maintain a custom solution, building from the ground up can be a great option as you’ll be left with something that exactly matches your needs and has the flexibility to morph as your needs change.
While the lift to create a robust, flexible, and maintainable solution from scratch is not rocket science, it can be a sizable investment. Sometimes, your engineering resources are better spent elsewhere.
Verdict: Build your account system custom if you have the experience and engineering resources to do so. It will give you the greatest amount of flexibility as your game evolves over time. Otherwise, look for a third party solution that will most closely fit your needs.
Third Party Centralized Accounts
Sometimes your hand will be forced even if you do have an experienced team that would like to build a custom system. For example, if you are working with a publisher that has a central technology platform, it may be a requirement for your studio to ship your game utilizing their account system. Examples here include EA, 2K, and Epic Games.
In other scenarios, choosing a third-party solution may be the best path forward for the game you’re building as your requirements are straightforward and you would prefer to invest your engineering effort into other problems.
The authentication providers outlined in part one also provide account systems. Ones that you might be interested in utilizing are:
Catena Tools
Wolfjaw’s own toolset, Catena Tools, provides auth and accounts functionality out of the box. It is meant to be extensible, giving you the same flexibility as if you had written a custom solution, while giving you a jump start to the feature set. If you or your team would like assistance standing Catena up on your infrastructure contact our development team or join our Discord. We’d be happy to help!
FusionAuth - https://fusionauth.io/
FusionAuth is a centralized authentication and accounts service that provides integrations with identity providers in the gaming industry. One of the largest factors to consider when choosing whether to build your own authentication and account system is cost. Many third party vendors that are focused entirely on auth and accounts have a considerable price tag attached to them, especially as your player counts rise. FusionAuth is no exception.
Epic Online Services (EOS) - https://dev.epicgames.com/en-US/services
EOS is a games industry specific toolset that is free to use and can be implemented in a white-labeled fashion. If you’d like to build the rest of your backend custom, their connect interface can be used in isolation for authentication and account management. They have the added bonus of providing Kid Web Services (KWS) which can be used in tandem with their auth/accounts offering for handling players that are underage. One constraint of using EOS is they do not provide an off the shelf method to link different identity providers to a single player’s account, rather providing a series of APIs to accomplish that task. If you plan to support cross-progression (allowing a player to log in to their same account on multiple platforms), you will need to write a backend service to sit in between game clients and EOS that facilitates an account linking flow similar to what is outlined earlier in this post.
Epic Account Services (EAS) - https://dev.epicgames.com/en-US/services-account
EAS, which is commonly confused with EOS, is worth calling out as an option if you’d like to wholly shift the onus of account management to Epic. It is a specific subset of EOS that is not able to be implemented in a white-labeled fashion. EAS allows you to let players use their Epic Games accounts with your game. You get the added benefit of account linking (linking multiple identity providers to a single central account) and social features out of the box. The main reason a studio may want to shy away from using EAS is because they don’t want to be dependent on Epic’s ecosystem or have Epic branding in their game in any way.
Pragma, RallyHere, Nakama, AccelByte, Playfab
There are a variety of other solutions on the market today, that provide auth and accounts functionality. Each of these platforms provides additional features on top of auth and accounts, and each has their own strengths and weaknesses. For the purposes of this post, we will not dive into the nuances of each platform.
Verdict: If you don’t have the engineering resources or know-how to build an account system from scratch, a third party provider can be a great option. Each has their pros and cons, so you should ask yourself what’s important to your studio. Where on the spectrum are your needs with regards to convenience vs. control? How much ownership do you want to have over the system?
Compliance
Wolfjaw studios is not a law firm and none of the following is legal advice. Instead, it is a high level overview of the technical implications of two major laws that will impact the engineering effort that your studio will need to exert if you choose to build a custom account system. Consult legal counsel for more information about legal implications for your locale.
General Data Protection Regulation (GDPR)
GDPR is a European Union law that regulates the usage of personal data of EU citizens or residents. While it only applies to players of your game from the EU, it is best practice to be in compliance for all of your players’ personal data.
First off, you will need to understand the concept of personal identifiable information or PII. This is data that when used alone or with any other relevant information, can identify an individual. This data must be stored in a secure way, such as an encrypted database, and should not make its way into places such as centralized logging systems.
With that understanding, you will need to build the following features:
- TOS and EULA agreement upon account registration
- An automated way for users to request and receive an export of their PII (Right Of Access By The Data Subject)
- An automated way for users to request a deletion of their PII (Right To Be Forgotten)
Children’s Online Privacy Protection Rule (COPPA)
COPPA is a US law that enforces regulations concerning children’s online privacy. Some companies circumvent COPPA by providing an “I am older than 13” checkbox during account creation, though because we’re in the games industry and kids love games we have an obligation to provide the safest experiences possible.
To allow kids under the age of 13 to play your game, you will need to build a system that provides verifiable parental consent, or VPC. While this can be done custom, due to its complexity we highly recommend leaning on a third party solution for this. Options include Kids Web Services and k-ID.
Conclusion
While account systems are not the most complex pieces of software in games, there is a significant amount of work that needs to be invested into them to make them easy to interact with, robust, secure, and safe. In future posts we will dive deeper into specific facets of building account systems, providing code examples alongside for you to reference. Additionally, we will be providing more posts like this to give high level overviews of the different components of a game backend.
If you would like to circumvent the need to build an accounts system from scratch, consider using Catena Tools. If you or your team would like assistance standing Catena up on your infrastructure contact our development team or join our Discord. We’d be happy to help!